In software development, writing functional code is just the beginning. The real challenge — and arguably the more important one — is writing clean code. Clean code is easy to read, maintain, and enhance, forming the backbone of successful software projects. It enables seamless collaboration among developers and ensures the longevity of the codebase.
When maintaining a codebase, keeping up with the latest technologies is just as important as clean coding practices. For instance, if you’re considering migrating from Java 11 to Java 17, adopting clean code principles can make the transition smoother. Cleaner, well-structured code is easier to refactor and adapt to the changes and new features introduced in newer Java versions.
In this blog, we’ll dive into the essence of clean code, why it’s critical, and how to apply its principles effectively in your Java projects.
What is clean code?
Clean code goes beyond mere functionality. It’s code that is intuitive, maintainable, and structured for future growth. As Robert C. Martin, author of Clean Code: A Handbook of Agile Software Craftsmanship, eloquently states, “Clean code is simple and direct. Clean code reads like well-written prose.”
When you write clean code, you’re not just writing for the compiler — you’re writing for other developers, including your future self. Clean code embodies the following attributes:
- Readable: It’s clear and easy to understand at a glance.
- Maintainable: It can be updated or extended with minimal risk of breaking existing functionality.
- Testable: Its structure supports seamless creation of automated tests.
Key principles of clean code
Clean code is essential for creating software that is easy to read, maintain, and scale. When working on tasks like migrating from Java 11 to 17, following clean code principles becomes even more critical to ensure the transition is smooth and the updated code remains efficient and understandable. Below are the fundamental principles to keep your code in top shape:
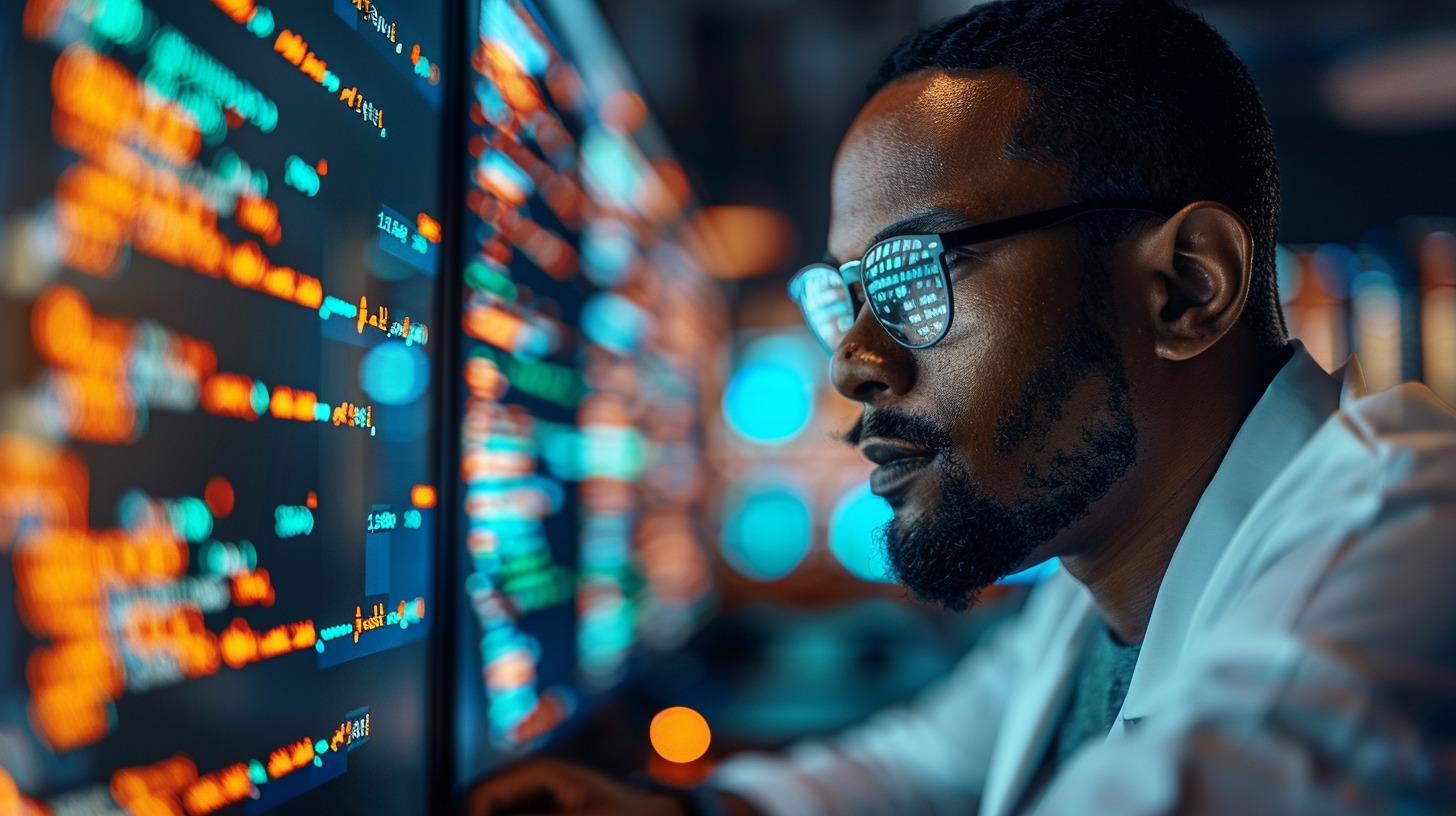
1. Use meaningful names
Names in your code should clearly convey purpose. Choose descriptive names for variables, functions, and classes, avoiding cryptic abbreviations or generic terms like temp or data. A well-named element makes the code self-explanatory.
“`java
// Bad
int d; // elapsed time in days
// Good
int elapsedTimeInDays;
“`
2. Keep functions small
Functions should do one thing and do it well. If a function handles multiple tasks, break it into smaller, focused functions. Aim for brevity — ideally between 5 to 15 lines of code.
“`java
// Bad
void saveUserData() {
// validate input
// save to database
// send confirmation email
}
// Good
void saveUserData() {
validateInput();
saveToDatabase();
sendConfirmationEmail();
}
“`
3. Avoid magic numbers and strings
Hard-coded values (often called “magic numbers” or “magic strings”) are cryptic and reduce clarity. Replace them with named constants to enhance readability and maintainability.
“`java
// Bad
double circleArea = radius * radius * 3.14159;
// Good
final double PI = 3.14159;
double circleArea = radius * radius * PI;
“`
4. Use comments wisely
Comments should provide context or explain why a certain choice was made, not what the code does. Ideally, your code should be self-explanatory through clean naming and structure.
“`java
// Bad
// increment i by 1
i++;
// Good
// Retry request if the first attempt fails due to network issues
retryRequest();
“`
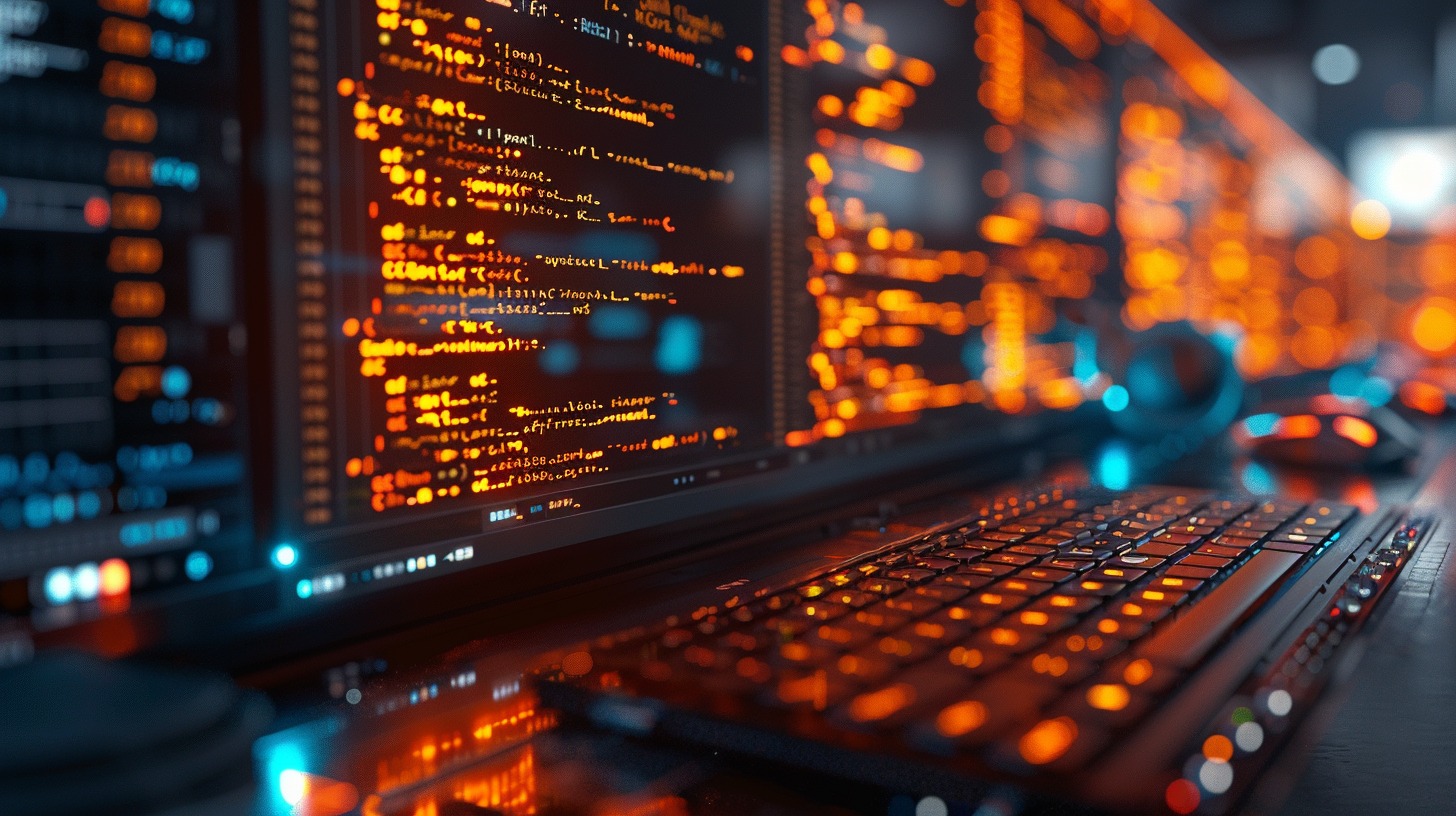
5. Maintain consistent formatting
Consistency in formatting enhances readability and creates a unified codebase. Use proper indentation, spacing, and line breaks. Adopt a style guide, such as Google’s Java Style Guide, to ensure consistency across your project.
“`java
// Bad
if(isValid){processData();}
// Good
if (isValid) {
processData();
}
“`
6. Handle errors gracefully
Effective error handling is a key aspect of clean code. Avoid using exceptions for routine flow control, and strive to provide meaningful error messages. Failing fast by validating inputs early is also a good practice.
“`java
// Bad
try {
// code that might throw an exception
} catch (Exception e) {
// handle exception
}
// Good
if (input == null) {
throw new IllegalArgumentException(“Input cannot be null”);
}
“`
Why clean code matters
Clean code is not just about aesthetics — it delivers practical benefits that can significantly impact a project’s success:
- Simplifies maintenance: Clean code reduces the time spent debugging and implementing new features. Developers can quickly understand and modify it without introducing errors.
- Boosts team collaboration: In a team setting, clean code ensures everyone can easily read and navigate the codebase, minimizing miscommunication and enhancing productivity.
- Reduces technical debt: Clean code minimizes the accumulation of technical debt, making it easier to scale and adapt the codebase over time while reducing future development costs.